Top 7 Benefits of Hierarchical Inheritance in Java
Hierarchical inheritance in Java allows multiple child classes to inherit from a single parent class, promoting code reusability, modularity, and scalability. It simplifies maintenance, supports the DRY principle, and enables specialization and polymorphism. This approach ensures cleaner, more organized code, making it easier to develop, debug, and extend applications efficiently.
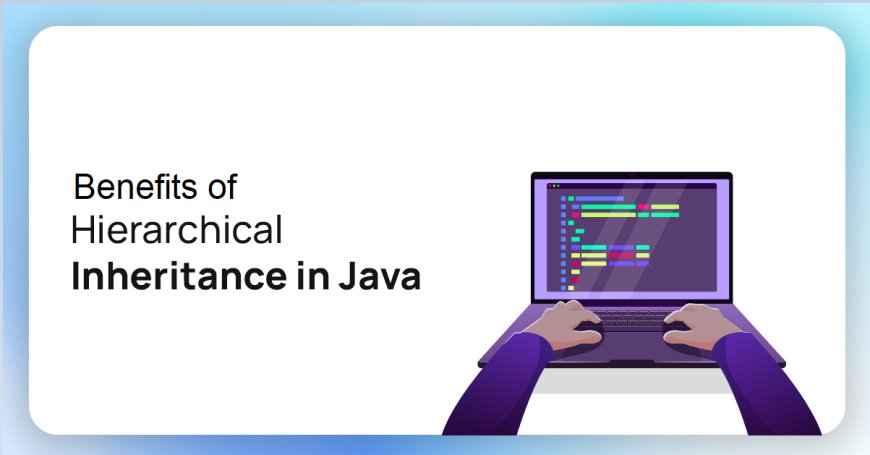
Hierarchical inheritance is a fundamental concept in object-oriented programming, where multiple child classes derive from a single parent class. This structure is widely used in Java to streamline development, promote reusability, and maintain an organized codebase. Here, we’ll explore the top seven benefits of hierarchical inheritance, highlighting how it enhances efficiency, scalability, and maintainability in software development.
Code Reusability
One of the most significant advantages of hierarchical inheritance is its ability to reuse code effectively. When common attributes and behaviors are defined in the parent class, all child classes automatically inherit them. This eliminates the need to duplicate functionality across multiple classes, saving time and reducing errors.
For instance, if a parent class defines general features, such as logging or basic calculations, all child classes can leverage these functionalities without implementing them repeatedly. This not only enhances consistency but also simplifies future updates or modifications.
Modular and Scalable Code
Hierarchical inheritance helps create modular code by clearly separating shared functionalities and specialized behaviors. Each child class focuses on its unique features while inheriting the common traits of the parent class. This design makes the system more scalable, as new functionalities can be added by introducing additional child classes without altering the existing structure.
This modular approach is particularly valuable in large projects, as it ensures that each component remains independent yet seamlessly integrated with the overall framework.
Easy Maintenance
Maintaining and updating code becomes significantly easier with hierarchical inheritance. If a change is needed in shared functionality, it can be made directly in the parent class. This update is automatically reflected across all child classes, ensuring consistent behavior throughout the application.
For example, if an application requires a new security feature, adding it to the parent class instantly extends the functionality to all child classes, simplifying the process and reducing potential errors.
Promotes the DRY Principle
The Don’t Repeat Yourself (DRY) principle is a cornerstone of efficient programming, and hierarchical inheritance adheres to it by centralizing shared logic. By defining common features in the parent class, developers avoid duplicating code across multiple child classes, leading to a cleaner and more organized codebase.
This principle not only reduces redundancy but also minimizes the risk of bugs, as any corrections or enhancements are made in a single place, benefiting all related components.
Encourages Specialization
Hierarchical inheritance enables child classes to specialize in their specific functionalities while sharing common behaviors with other classes. This fosters the creation of clear, domain-specific classes that are easy to understand and manage.
For example, in a system with a shared base class, each subclass can focus on implementing its unique characteristics. This specialization ensures that the overall code remains organized, promoting efficiency and clarity.
Supports Polymorphism
Polymorphism, a key feature of object-oriented programming, is naturally supported by hierarchical inheritance. It allows a single interface to represent different forms of behavior, making the system more dynamic and flexible.
For instance, a parent class can serve as a common type for multiple child classes, enabling developers to write code that works seamlessly with objects of different child classes. This capability is especially useful in scenarios where runtime behavior needs to adapt dynamically based on specific conditions.
Simplifies Debugging
Debugging and troubleshooting are streamlined in systems that use hierarchical inheritance. By centralizing shared functionalities in the parent class, developers can easily identify and resolve issues without having to examine each child class individually.
For instance, if a common method in the parent class requires optimization or correction, fixing it in one place ensures that all related child classes benefit from the update. This approach saves time and ensures consistency across the application.
Additional Considerations
While hierarchical inheritance offers numerous advantages, it’s essential to use it thoughtfully to avoid potential challenges:
-
Clarity in Design: Ensure that the relationship between parent and child classes is logical and adheres to a clear "is-a" structure. Overuse of inheritance can lead to complex and tightly coupled code.
-
Alternatives When Needed: In scenarios where hierarchical relationships are not appropriate, consider composition or other design patterns to achieve a more suitable structure.
Conclusion
Hierarchical inheritance in Java is a powerful tool that enables developers to build efficient, scalable, and maintainable software. By promoting code reusability, modularity, and adherence to the DRY principle, it simplifies development and ensures consistency across applications. Furthermore, it encourages specialization, supports polymorphism, and makes debugging more straightforward.
When used correctly, hierarchical inheritance can transform a project, making it easier to manage and extend as requirements evolve. Whether you’re developing a small utility or a complex enterprise system, leveraging hierarchical inheritance can lead to cleaner, more effective code that stands the test of time.
What's Your Reaction?
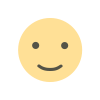

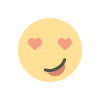

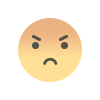
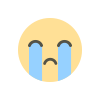
