Laravel Development Critical Security Parameters to Monitor
Discover security parameters to monitor in Laravel development to protect your applications from vulnerabilities and cyber threats.
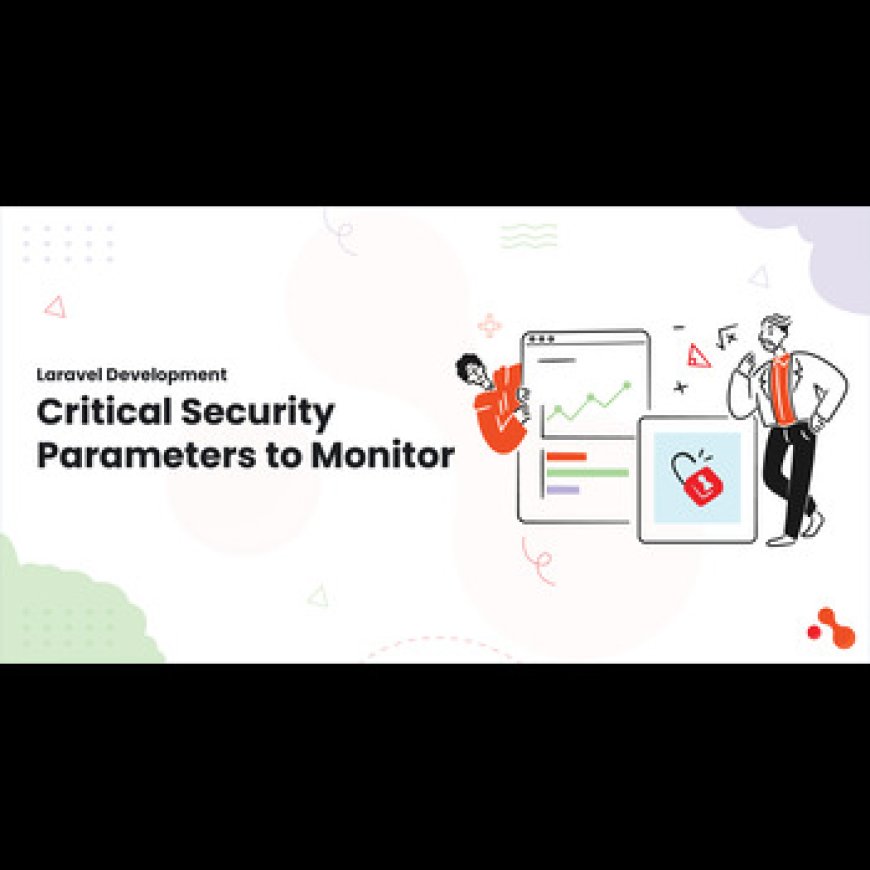
Introduction
Laravel, a popular PHP framework renowned for its elegance and robust feature set, has become a staple in web application development. While Laravel offers numerous benefits, security remains a crucial aspect that developers must prioritize. Effective monitoring of critical security parameters is essential to protect Laravel applications from vulnerabilities and cyber threats.
Laravel, known for its elegant syntax and robust features, also emphasizes security within its ecosystem. However, no framework is fully secure especially with the high level of customization. This article provides an in-depth examination of the key security parameters that require vigilant monitoring in Laravel development.
Security Protocols in Laravel Applications
Laravel, a widely used PHP framework, comes with robust security features to help developers build secure web applications. However, leveraging these features effectively requires understanding the various security protocols that Laravel supports. This ensures that applications are protected from common vulnerabilities and threats. Below are some key security protocols and Laravel best practices that developers should implement in applications.
- Authentication and Authorization.
- CSRF Protection (Cross-Site Request Forgery).
- XSS Protection (Cross-Site Scripting)
- SQL Injection Prevention
- Password Hashing
- HTTPS and Secure Cookies
- Rate Limiting
- Logging and Monitoring
- Validation and Sanitization
- File Upload Security
- Content Security Policy (CSP)
- Session Security
- Access Control and Role-Based Access
- Dependency Management and Security Patches
Security is a crucial aspect of Laravel applications, and the framework provides several in-built protocols and best practices to secure web applications. It is vital developers make the most of these built-in features however, customization is not uncommon in Laravel applications. In this case it is essential to make an extra effort to secure the application.
Security Parameters to Monitor
To ensure the security of a Laravel application, it's important to continuously monitor various security parameters. Here are the key security parameters you should monitor:
- Failed Login Attempts: Monitoring failed login attempts can help you detect brute-force attacks or suspicious login activity. Implement logging and alerts to track repeated failed login attempts within a short time frame.
- How to Monitor: Use Laravel’s built-in authentication system and middleware like throttle to limit login attempts and track failures. You can log each failed attempt using Laravel's logging system.
- Example:
php code
if (!Auth::attempt($credentials)) {
Log::warning('Failed login attempt for user: '.$credentials['email']);
}
- Suspicious IP Activity: Monitor the IP addresses accessing your application, especially for unusual geographic locations or IPs known for malicious activity. Implement IP blocking or rate limiting for repeated suspicious requests.
- How to Monitor: Use Laravel’s Request class to capture IP addresses and log or block suspicious ones.
php code
$ipAddress = $request->ip();
Log::info('Access from IP: ' . $ipAddress);
- Unusual User Behavior: Track user activities, such as frequent password resets, account lockouts, or unexpected actions, which may indicate an account compromise or suspicious behavior.
- How to Monitor: Log important user actions, such as password changes, access to restricted resources, or frequent use of critical features.
php code
Log::info('User ' . Auth::user()->email . ' reset their password.');
Access to Sensitive Data
Ensure that access to sensitive information, such as personally identifiable information (PII) or financial records, is logged and restricted based on user roles and permissions.
- How to Monitor: Use Laravel's built-in authorization and policy system to ensure sensitive data is only accessed by authorized users and log each access.
- Example:
php
Copy code
if (Gate::allows('view-sensitive-data')) {
Log::info('Sensitive data viewed by user: ' . Auth::user()->email);
}
- Unusual File Uploads: Monitoring file uploads is crucial, as malicious files could be uploaded to compromise the system. Ensure that file types and sizes are restricted, and monitor any unusual file uploads.
- How to Monitor: Log each file upload and inspect for suspicious files, especially non-standard formats or scripts.
php code
Log::info('File uploaded by user: ' . Auth::user()->email . ', File: ' . $fileName);
- Failed Login Attempts: Monitoring failed login attempts can help you detect brute-force attacks or suspicious login activity. Implement logging and alerts to track repeated failed login attempts within a short time frame.
- How to Monitor: Use Laravel’s built-in authentication system and middleware like throttle to limit login attempts and track failures. You can log each failed attempt using Laravel's logging system.
- Example:
php code
if (!Auth::attempt($credentials)) {
Log::warning('Failed login attempt for user: '.$credentials['email']);
}
Suspicious IP Activity
Monitor the IP addresses accessing your application, especially for unusual geographic locations or IPs known for malicious activity. Implement IP blocking or rate limiting for repeated suspicious requests.
- How to Monitor: Use Laravel’s Request class to capture IP addresses and log or block suspicious ones.
php code
$ipAddress = $request->ip();
Log::info('Access from IP: ' . $ipAddress);
- Unusual User Behavior: Track user activities, such as frequent password resets, account lockouts, or unexpected actions, which may indicate an account compromise or suspicious behavior.
- How to Monitor: Log important user actions, such as password changes, access to restricted resources, or frequent use of critical features.
php code
Log::info('User ' . Auth::user()->email . ' reset their password.');
- Access to Sensitive Data: Ensure that access to sensitive information, such as personally identifiable information (PII) or financial records, is logged and restricted based on user roles and permissions.
- How to Monitor: Use Laravel's built-in authorization and policy system to ensure sensitive data is only accessed by authorized users and log each access.
- Example:
php
Copy code
if (Gate::allows('view-sensitive-data')) {
Log::info('Sensitive data viewed by user: ' . Auth::user()->email);
}
- Unusual File Uploads: Monitoring file uploads is crucial, as malicious files could be uploaded to compromise the system. Ensure that file types and sizes are restricted, and monitor any unusual file uploads.
- How to Monitor: Log each file upload and inspect for suspicious files, especially non-standard formats or scripts.
php code
Log::info('File uploaded by user: ' . Auth::user()->email . ', File: ' . $fileName);
- Cross-Origin Resource Sharing (CORS): CORS policies should be properly configured to prevent unauthorized domains from making API requests. Monitor for any misconfigurations or unauthorized requests from external domains.
- How to Monitor: Use Laravel’s CORS package to manage and monitor CORS policy violations.
Application Debug Mode Status
- Parameter: APP_DEBUG in the .env file.
- Why Monitor: When debug mode is enabled (APP_DEBUG=true), detailed error messages and stack traces are displayed, potentially revealing sensitive information to attackers. In production environments, this should always be set to false.
- How to Monitor: Regularly check the .env file and ensure that APP_DEBUG is set to false in production. Implement automated checks as part of your deployment process to prevent enabling debug mode in production accidentally.
Environment Configuration File Access
- Parameter: Access permissions for the .env file.
- Why Monitor: The .env file contains sensitive configuration details, such as database credentials, API keys, and encryption keys. Unauthorized access to this file can lead to security breaches.
- How to Monitor: Ensure the .env file has the correct file permissions (typically 644), making it readable only by the application server and not accessible to the public. Use server configuration settings to deny access to the .env file directly.
- Database Configuration and Access
- Parameter: Database connection details (DB_HOST, DB_PORT, DB_DATABASE, DB_USERNAME, DB_PASSWORD).
- Why Monitor: Secure database configurations prevent unauthorized access to the database. Weak or exposed database credentials can lead to data breaches.
- How to Monitor: Use strong, complex passwords for database users. Regularly rotate credentials and ensure that only the necessary services and IP addresses have access to the database. Monitor database connections for unusual or unauthorized access attempts.
Cross-Site Request Forgery (CSRF) Protection
- Parameter: CSRF token validation in forms.
- Why Monitor: CSRF attacks can force authenticated users to perform unwanted actions without their consent. Laravel’s CSRF protection ensures that every form submission is accompanied by a unique token.
- How to Monitor: Ensure CSRF protection is enabled by default through the web middleware group. Regularly audit forms to verify the inclusion of CSRF tokens using the @csrf Blade directive.
Cross-Site Scripting (XSS) Protection
- Parameter: Output escaping and input validation.
- Why Monitor: XSS attacks inject malicious scripts into web pages viewed by other users. Proper escaping of output and sanitization of input prevent such attacks.
- How to Monitor: Use Laravel’s {{ }} syntax to automatically escape data when rendering views. Implement input validation using Laravel’s validation rules to sanitize user input before processing.
Rate Limiting Configuration
- Parameter: Rate limiting settings for routes and API endpoints.
- Why Monitor: Rate limiting helps protect against brute force attacks, denial of service (DoS) attacks, and abuse of APIs by limiting the number of requests a user can make.
- How to Monitor: Configure and regularly review the ThrottleRequests middleware settings in routes/api.php or routes/web.php to ensure appropriate rate limits are in place.
Authentication and Authorization
- Parameter: User authentication and role-based access control.
- Why Monitor: Proper authentication and authorization ensure that only authorized users can access specific resources or perform particular actions. Weak authentication can lead to unauthorized access.
- How to Monitor: Regularly audit user authentication mechanisms (e.g., passwords, OAuth tokens) and review user roles and permissions defined in policies and gates. Use Laravel’s built-in authentication and authorization features to manage user access effectively.
Session Management
- Parameter: Session handling configuration (SESSION_DRIVER, SESSION_LIFETIME, SESSION_SECURE_COOKIE).
- Why Monitor: Secure session management prevents session hijacking and unauthorized access. Insecure session handling can lead to compromised user accounts.
- How to Monitor: Set the session driver to secure, configure session lifetimes appropriately, and enable SESSION_SECURE_COOKIE to ensure that session cookies are only transmitted over secure HTTPS connections.
Encryption Key and Protocols
- Parameter: APP_KEY in the .env file and encryption protocols.
- Why Monitor: The encryption key (APP_KEY) is critical for securing encrypted data and sessions. If compromised, encrypted data can be exposed.
- How to Monitor: Regularly verify that the APP_KEY is set and secure. Use Laravel’s artisan key:generate command to generate a strong encryption key. Monitor usage of secure protocols like HTTPS for data transmission.
Logging and Monitoring
- Parameter: Logging configuration (LOG_CHANNEL, LOG_LEVEL) and log file access.
- Why Monitor: Proper logging helps detect suspicious activities and security incidents. Logs should be securely stored and accessible only to authorized personnel.
- How to Monitor: Configure the logging level to capture critical security-related events without exposing sensitive information. Use centralized logging solutions and regularly review logs for signs of unauthorized access or suspicious activity.
Third-Party Package Security
- Parameter: Dependencies listed in composer.json and composer.lock.
- Why Monitor: Third-party packages may introduce vulnerabilities. Regular updates ensure that security patches are applied promptly.
- How to Monitor: Use tools like composer audit to check for known vulnerabilities in third-party packages. Regularly update dependencies using composer update and remove unused packages.
Security Headers
- Parameter: HTTP security headers (e.g., Content-Security-Policy, X-Content-Type-Options, X-Frame-Options, Strict-Transport-Security).
- Why Monitor: Security headers protect against various attacks like XSS, clickjacking, and MIME-type sniffing.
- How to Monitor: Implement and monitor security headers using Laravel middleware or server configuration. Use online tools to test header implementation and effectiveness.
Hire Laravel Developers
It is vital for businesses to incorporate all the correct security measures in their application. The best way to accomplish this is by choosing the best Laravel development company.
Acquaint Softtech is a software development outsourcing company in India with over 10 years of experience. You can trust us to deliver secure Laravel solutions.
When you hire remote developers from here, you can be confident of the security of your Laravel application. We have already delivered over 5000 applications worldwide.
Conclusion
Monitoring critical security parameters in Laravel development is not just about implementing tools but also about fostering a culture of security awareness and proactive management. As we've seen from various case studies, robust security practices not only prevent breaches but also build foundational trust with users, making security a cornerstone of successful Laravel applications.
Moving forward, developers must stay updated with emerging security trends and Laravel's evolving features to maintain secure and resilient applications. The security of Laravel applications is an ongoing process that requires constant vigilance and adaptation to emerging threats.
By studying successful case studies and monitoring critical security parameters, developers can fortify their applications against potential vulnerabilities. Embracing a culture of security within the Laravel community will contribute to the development of more resilient and trustworthy applications.
Take advantage of the Laravel development services of the professionals at Acquaint Softtech to gain an upper edge.
What's Your Reaction?
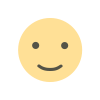

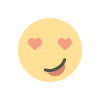

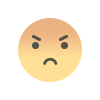
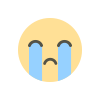
