Real-World Lessons on Enhancing Code Flexibility in Laravel
Discover practical tips and strategies for improving code flexibility in Laravel development. Learn to enhance code maintainability.
Introduction
Laravel is the framework of choice for many developers due to its elegant syntax, robust features, and vibrant ecosystem. There is a very good reason why. It happens to be one of the most secure frameworks that allows one to build robust solutions.
However, code inflexibility is an issue that many types of applications tend to face when attempting to scale. Enhancing Laravel code flexibility involves using design principles and architectural practices that allow your code to adapt to changing requirements, new features, and various business scenarios.
This article dives deep into real-world strategies for enhancing code flexibility in Laravel, drawing on lessons from seasoned developers and successful projects.
Consequences of Code Inflexibility in Laravel
Code inflexibility refers to situations where a codebase becomes hard to change, scale, or extend. This can happen due to tightly coupled components, over-reliance on specific patterns, or monolithic architecture.
Code inflexibility in Laravel (or any framework) can have significant consequences that hinder the application's scalability, maintenance, and adaptability.
Inflexibility in Laravel code restricts an application's ability to grow and adapt, ultimately slowing down development and adding long-term risks and costs. Here are some of the key impacts of inflexible code:
Increased Maintenance Time and Costs
Maintenance becomes more time-consuming and costly, as developers have to spend extra time understanding dependencies and rewriting large sections of code.
Complexity in Introducing New Features
Introducing new features becomes risky and complex, delaying deployment timelines and increasing the likelihood of bugs.
Performance Degradation and Scalability Challenges
As the application grows, performance can degrade, and it becomes challenging to modify the architecture to handle additional load or new functionality.
Increased Risk Due to Inadequate Testing
The inability to conduct proper testing increases the risk of deploying buggy code, leading to a lower-quality product and more time spent fixing issues after release.
Lower Productivity and Developer Frustration
Developers take longer to understand the codebase and implement changes, leading to lower productivity and frustration, which can even impact team morale.
Higher Likelihood of Bugs with Updates
This increases the likelihood of introducing bugs when making updates, as dependencies are harder to track and manage.
Lack of Reusable Components
Reusable components save time and effort; without them, developers have to rewrite similar code across the codebase, leading to code duplication and inconsistency.
Decline in Code Readability
As code readability declines, onboarding new developers takes longer, and it becomes harder for team members to understand the existing code, slowing down the overall development process.
Difficulty Implementing Laravel Best Practices
The team may struggle to implement Laravel best practices, such as event-driven architecture, containerization, or cloud adoption, as inflexible code is not designed for modularity or easy integration with new technologies.
Negative User Experience and Brand Impact
Users may experience a slower, less responsive application that does not meet their needs, leading to decreased satisfaction, lower retention, and potentially damaging the brand’s reputation.
Security Risks and Compliance Issues
Security flaws can remain unresolved for longer, putting user data and application integrity at risk, which can lead to compliance issues, fines, and reputational damage.
Applying best practices, such as dependency injection, SOLID principles, and modular architecture, can help ensure the code remains adaptable, scalable, and maintainable over time. It will work in your favor to trust a professorial Laravel development company like Acquaint Softtech.
We are a well-established software development outsourcing company in India with over 10 years of experience. Our developers always implement best practices and take all necessary measures to prevent code inflexibility.
Real-World Case Studies
eCommerce Platform Scalability
An eCommerce platform developed in Laravel faced challenges as it scaled. Implementing the Repository pattern, event sourcing, and strategically breaking down the application into microservices helped resolve issues related to scalability and performance.
eCommerce Platform
An eCommerce platform built on Laravel needed to integrate multiple payment gateways to cater to different customer preferences. The team created a PaymentGatewayInterface and implemented it for each payment provider. This allowed them to switch payment providers without changing the core business logic. The platform could easily add or remove payment gateways as needed, improving flexibility and reducing the time required for future integrations.
SaaS Application Development
A Software as a Service (SaaS) application leveraging Laravel implemented a multi-tenant architecture with dedicated services for billing, user management, and feature control. This modular approach facilitated independent scaling and updating of different parts of the system.
Content Management System (CMS)
A Laravel-based CMS is needed to support multiple content types and allow for easy customization by users. The team used a combination of polymorphic relationships and a flexible content model to allow users to create new content types without modifying the core codebase. The CMS became highly flexible, enabling users to create and manage various content types easily. The architecture allowed for rapid feature development and customization.
Social Media Application
A social media application built with Laravel needed to scale quickly and accommodate new features like messaging, notifications, and user tagging. The team implemented Laravel's event broadcasting to handle real-time notifications and messaging. This decoupled the notification logic from the core application, allowing for easier updates and changes. The application was able to scale effectively, and new features could be added with minimal disruption to existing functionality.
Educational Platform
An educational platform is needed to support various types of courses, quizzes, and user interactions while allowing instructors to customize their content. The team created interfaces for different content types and implemented them in various classes. This allowed instructors to create new content types without modifying the core application. The educational platform became highly flexible, allowing instructors to create and manage diverse content types easily. The architecture facilitated rapid development and testing.
Automated Testing for Healthcare Compliance
A healthcare SaaS provider built a Laravel application to manage patient data and scheduling. The development team built automated unit and integration tests into the Laravel application using dependency injection and repository patterns to make the code testable. The ability to test each component in isolation helped the team ensure it complied with strict healthcare regulations. It ensures the code is flexible enough to adapt to changes and every change to the code is thoroughly tested.
Scalable Content Management System with Dependency Injection
A digital media agency needed a custom content management system (CMS) to handle multiple high-traffic websites. The team implemented dependency injection across the application to make it easier to configure different content modules and services per client. This allowed them to provide custom configurations for each client without duplicating code and switching between data storage systems.
Microservices Migration for a Fintech Application
A fintech startup initially built its transaction management system in a monolithic Laravel application. The team gradually refactored the monolithic Laravel app into smaller, independent services. They used Laravel’s service providers and repository patterns to extract functionalities as services, each handling a specific domain. They transitioned to microservices architecture making it easy to add features and accommodate growth without reworking the entire codebase.
Flexible Multi-Tenant SaaS Platform with Event Broadcasting
A SaaS company developed a project management tool for multiple businesses, each with different workflows and needs. Laravel’s event broadcasting and notification system allowed the team to create a highly customizable notification module. The flexible architecture allowed them to accommodate new clients with custom workflows without major code changes.
Rapid Feature Deployment in a Real-Time Social Media Platform
A social media startup wanted a Laravel-based platform to support real-time interactions and frequent updates. The development team adopted the SOLID principles to ensure Laravel code flexibility creating interfaces for key services and using dependency injection to swap implementations as needed. With modular components and a robust job-handling system, the team could roll out new features and scale the app efficiently.
Reusability in an Educational Platform for a Non-Profit
A non-profit organization used Laravel to build an educational platform for online courses and learning resources. The team employed a modular approach, organizing the codebase by features. The non-profit saved time and reduced costs by reusing modules across applications. They were able to scale and reach a broader audience without needing to start each project from scratch by maximizing code reusability.
Laravel Best Practices To Follow
- Use Service Providers Wisely: Service providers are the backbone of Laravel's dependency injection and service container. Use them to bind classes to interfaces, allowing for easier swapping of implementations.
- Leverage Dependency Injection: Instead of using facades or static calls, inject dependencies into your classes. This makes testing easier and promotes loose coupling.
- Follow the Repository Pattern: The repository pattern abstracts data access, making it easier to change data sources or implement caching.
- Utilize Interfaces and Contracts: Define interfaces for your services and use them in your code. This allows you to implement different versions of a service without changing the code that relies on it.
- Adopt Event-Driven Architecture: Use events and listeners to decouple components of your application. This allows you to add new features without modifying existing code.
- Configuration and Environment Variables: Keep your configuration flexible by using environment variables and configuration files. This allows for easy changes across different environments (development, staging, production).
- Version Your APIs: As your application evolves, your API might need to change. Versioning your APIs allows you to introduce new features without breaking existing clients.
- Embrace Testing: Writing tests help ensure that your code remains flexible and that changes do not introduce bugs.
- Implementing Interface-driven Design: Using interfaces can significantly decouple code and define clear contracts for what each component should implement. This approach not only makes the code more testable and robust but also easier to maintain and adapt to changing requirements.
- Using Patterns: Take advantage of patterns like Strategy, Adapter, and Decorator to dynamically change features.
- Event Sourcing: Event sourcing involves storing all changes to the application state as a sequence of events, giving applications immense flexibility.
- Queue System: Laravel’s queue system allows deferring time-consuming processes until a later time, thus enhancing application responsiveness and flexibility in managing workload.
Hire Laravel Developers
The case studies mentioned in this article serve the purpose of demonstrating the advantages of code flexibility in Laravel. They highlight its ease of maintenance, scalability and rapid adaptation. It also brings to light the importance to hire remote developers from a professional company.
Acquaint Softtech is one such company that offers a wide range of Laravel development services. We also happen to be an official Laravel Partner. Investing in code flexibility ultimately results in long-term efficiency, easier scalability, and the ability to adapt rapidly in competitive industries.
Conclusion
These case studies demonstrate how various strategies can enhance Laravel code flexibility. By adopting best practices and following the tips businesses can ensure the Laravel development company the hire creates applications that are easier to maintain, scale, and adapt to changes.
What's Your Reaction?
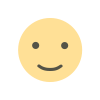

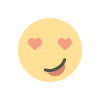

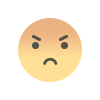
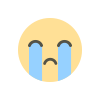
