What is the Use of Call by Value and Call by Reference in C?
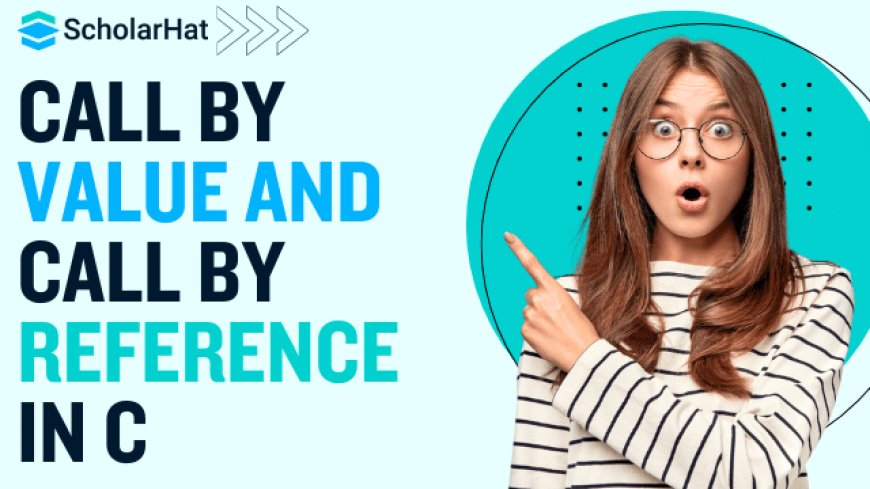
What is the Use of Call by Value and Call by Reference in C?
In the world of programming, understanding the nuances of different function calling mechanisms is essential for writing efficient and effective code. Two such mechanisms, call by value and call by reference in C, play a crucial role in C programming. This article delves deep into the uses of call by value and call by reference in C, exploring their differences, applications, and advantages.
Introduction to Call by Value and Call by Reference in C
In C programming, when a function is called, the way arguments are passed to that function can significantly impact the behavior and efficiency of the program. The two primary methods of passing arguments are call by value and call by reference. Both methods have their distinct uses and implications, which we will explore in detail. For a comprehensive guide on these concepts, refer to the C tutorial.
Understanding Call by Value
What is Call by Value?
Call by value is a method where the actual value of the argument is passed to the function. In this approach, any changes made to the parameter inside the function do not affect the original value outside the function. This method is straightforward and widely used in C programming.
How Call by Value Works
When a function is invoked, the actual parameters are copied into the formal parameters of the function. Any modifications to these formal parameters within the function do not alter the actual parameters.
Advantages of Call by Value
-
Simplicity: Call by value is simple to understand and implement.
-
Safety: Since the actual parameters are not modified, there is no risk of unintended side effects.
Examples of Call by Value
Here is an example to illustrate call by value in C:
c
Copy code
#include <stdio.h>
void swap(int x, int y) {
int temp;
temp = x;
x = y;
y = temp;
printf("Inside swap function: x = %d, y = %d\n", x, y);
}
int main() {
int a = 10, b = 20;
printf("Before swap function call: a = %d, b = %d\n", a, b);
swap(a, b);
printf("After swap function call: a = %d, b = %d\n", a, b);
return 0;
}
In this example, the values of a and b remain unchanged outside the swap function, demonstrating the concept of call by value.
Understanding Call by Reference
What is Call by Reference?
Call by reference is a method where the address of the actual parameter is passed to the function. This allows the function to modify the original value directly. Call by reference is powerful and useful when a function needs to update the original values.
How Call by Reference Works
When a function is called, the reference (or address) of the actual parameters is passed to the formal parameters. Consequently, any changes made to the formal parameters directly affect the actual parameters.
Advantages of Call by Reference
-
Efficiency: Call by reference avoids copying large amounts of data, making it more efficient for passing large structures or arrays.
-
Mutability: It allows the function to modify the original values, which can be essential for certain operations.
Examples of Call by Reference
Here is an example to illustrate call by reference in C:
c
Copy code
#include <stdio.h>
void swap(int *x, int *y) {
int temp;
temp = *x;
*x = *y;
*y = temp;
}
int main() {
int a = 10, b = 20;
printf("Before swap function call: a = %d, b = %d\n", a, b);
swap(&a, &b);
printf("After swap function call: a = %d, b = %d\n", a, b);
return 0;
}
In this example, the values of a and b are swapped successfully, demonstrating the concept of call by reference.
Comparing Call by Value and Call by Reference
Differences Between Call by Value and Call by Reference
-
Parameter Passing:
-
Call by Value: Passes a copy of the actual parameter.
-
Call by Reference: Passes the address of the actual parameter.
-
Effect on Actual Parameters:
-
Call by Value: Changes to the formal parameters do not affect the actual parameters.
-
Call by Reference: Changes to the formal parameters directly affect the actual parameters.
-
Efficiency:
-
Call by Value: Less efficient for large data as it involves copying.
-
Call by Reference: More efficient as it avoids copying large data.
-
Usage Scenarios:
-
Call by Value: Suitable for functions that do not need to modify the original data.
-
Call by Reference: Ideal for functions that need to update or modify the original data.
Applications of Call by Value and Call by Reference in C
When to Use Call by Value
-
Immutable Data: When the function should not alter the original data.
-
Simple Data Types: For passing basic data types like int, char, or float.
-
Function Safety: When you want to ensure that the function does not inadvertently modify the original values.
When to Use Call by Reference
-
Mutable Data: When the function needs to modify the original data.
-
Large Data Structures: For passing large arrays or structures to avoid the overhead of copying.
-
Efficiency: When performance is a concern and you want to avoid the cost of copying data.
Examples and Use Cases
Example 1: Call by Value for Simple Calculations
c
Copy code
#include <stdio.h>
int square(int x) {
return x * x;
}
int main() {
int num = 5;
int result = square(num);
printf("Square of %d is %d\n", num, result);
return 0;
}
In this example, call by value is used to pass a simple integer to a function that calculates its square.
Example 2: Call by Reference for Array Manipulation
c
Copy code
#include <stdio.h>
void reverseArray(int *arr, int size) {
int start = 0;
int end = size - 1;
while (start < end) {
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, size);
printf("Reversed array: ");
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
In this example, call by reference is used to reverse the elements of an array in place.
Benefits of Understanding Call by Value and Call by Reference
Enhanced Control Over Data Handling
Understanding the difference between call by value and call by reference gives programmers greater control over how data is handled within functions. This knowledge enables the selection of the most appropriate method for different scenarios.
Optimized Performance
By using call by reference for large data structures, programmers can optimize the performance of their programs, reducing the overhead associated with copying data.
Improved Code Maintainability
Using the correct parameter passing method enhances code readability and maintainability, making it easier for others to understand the intent and functionality of the code.
Conclusion
In conclusion, the use of call by value and call by reference in C plays a vital role in how functions interact with data. While call by value offers simplicity and safety, call by reference provides efficiency and mutability. Understanding these concepts is crucial for any C programmer aiming to write efficient, effective, and maintainable code.
By mastering these concepts, you will enhance your programming skills and be better equipped to tackle a wide range of programming challenges. Whether you are dealing with simple data types or complex data structures, knowing when to use call by value or call by reference will significantly improve the quality of your code. For more in-depth coverage of call by value and call by reference in C, explore the comprehensive call by value and call by reference in c tutorial. This resource provides additional examples and explanations to solidify your understanding and application of these important concepts in C programming.
By integrating these techniques into your programming repertoire, you can achieve greater control over data handling, optimize the performance of your programs, and ensure that your code is both robust and maintainable. Whether you are a beginner or an experienced programmer, mastering call by value and call by reference in C is an essential step towards becoming a proficient and versatile developer.
What's Your Reaction?
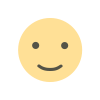

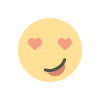

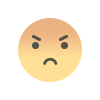
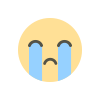
